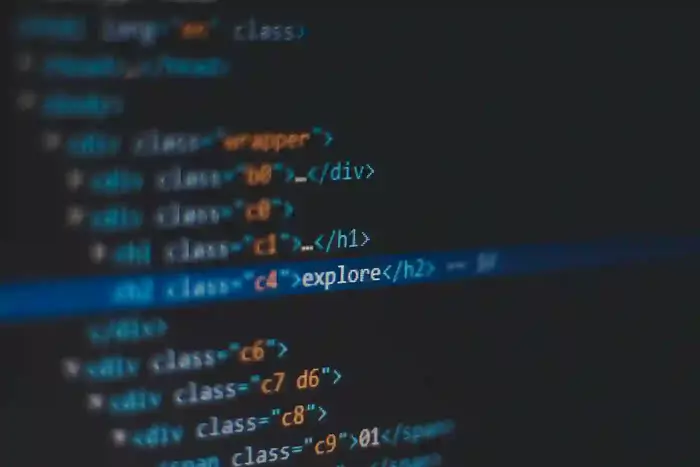
In this article we will talk about state management in ReactJs. I will try to explain 2-3 best state management libraries that you can try out & find the perfect one for you. For this article I have found state management libraries like Jotai, Recoil, and Redux . In this article, we will discuss the Jotai and Recoil libraries, and compare them with the widely used Redux library. We will also list the pros and cons of each library with different technologies.
Let’s talk about Jotai:
It is a relatively new state management library that is gaining popularity in the React community. Jotai uses a new approach to managing state in React that is based on atoms. An atom is a piece of state that can be shared between different components. Jotai makes it easy to create atoms, and these atoms can be composed to create more complex states.
Pros:
It has a small API surface area, which makes it easy to learn and use.
It uses a new approach to state management that is based on atoms, which makes it easy to share state between different components.
It provides a clean and concise way to manage state in React.
It is lightweight and has a small footprint.
It allows for better performance since it reduces the number of re-renders.
Cons:
It is a relatively new library and does not have as much community support as other state management libraries.
It may not be suitable for larger applications that require more complex state management.
It does not have built-in support for server-side rendering.
It has a smaller ecosystem than other state management libraries.
It may not be the best choice for applications that require time-travel debugging.
Example:
import { atom, useAtom } from 'jotai';
// Create an atom for the count state
const countAtom = atom(0);
// Component that uses the countAtom
function Counter() {
const [count, setCount] = useAtom(countAtom);
const increment = () => setCount((c) => c + 1);
const decrement = () => setCount((c) => c - 1);
return (
<div>
<button onClick={decrement}>-</button>
<span>{count}</span>
<button onClick={increment}>+</button>
</div>
);
}
Recoil:
It is another state management library that was developed by Facebook. Recoil provides a simple and intuitive API for managing state in React applications. It uses a new approach to state management that is based on atoms and selectors.
Pros:
It provides a simple and intuitive API for managing state in React applications.
It is lightweight and has a small footprint.
It has built-in support for server-side rendering.
It provides a way to manage asynchronous state.
It has a growing ecosystem of third-party packages.
Cons:
It is a relatively new library and does not have as much community support as other state management libraries.
It may not be suitable for larger applications that require more complex state management.
It does not provide time-travel debugging.
It may not be the best choice for applications that require performance optimization.
It does not provide built-in support for middlewares.
Example :
import { atom, useRecoilState } from 'recoil';
// Create an atom for the count state
const countAtom = atom({
key: 'countAtom',
default: 0,
});
// Component that uses the countAtom
function Counter() {
const [count, setCount] = useRecoilState(countAtom);
const increment = () => setCount((c) => c + 1);
const decrement = () => setCount((c) => c - 1);
return (
<div>
<button onClick={decrement}>-</button>
<span>{count}</span>
<button onClick={increment}>+</button>
</div>
);
}
Redux:
It is one of the most widely used state management libraries for React. It is based on the Flux architecture, and it provides a way to manage application state in a predictable and consistent way. It is a mature library with a large ecosystem and many third-party packages.
Pros:
It is a mature library with a large ecosystem and many third-party packages.
It provides time-travel debugging, which makes it easy to debug complex state management issues.
It has built-in support for middlewares, which allows for better performance optimization.
It is suitable for larger applications that require more complex state management.
It provides a way to manage asynchronous state with Redux-Saga.
Cons:
It has a steep learning curve and can be difficult to learn for beginners.
It has a larger API surface area than other state management libraries, which can make it more complex to use.
It can be verbose, which can make it harder to read and understand
Example :
import { createStore } from 'redux';
// Define the reducer for the count state
function counterReducer(state = { count: 0 }, action) {
switch (action.type) {
case 'INCREMENT':
return { ...state, count: state.count + 1 };
case 'DECREMENT':
return { ...state, count: state.count - 1 };
default:
return state;
}
}
// Create the Redux store
const store = createStore(counterReducer);
// Component that uses the count state from the Redux store
function Counter() {
const count = useSelector((state) => state.count);
const dispatch = useDispatch();
const increment = () => dispatch({ type: 'INCREMENT' });
const decrement = () => dispatch({ type: 'DECREMENT' });
return (
<div>
<button onClick={decrement}>-</button>
<span>{count}</span>
<button onClick={increment}>+</button>
</div>
);
}
By analyzing these examples, you can see how both Recoil and Jotai offer a simpler and more intuitive approach to state management in React, with less boilerplate code required to implement even basic state updates. However, there are some key differences between the two libraries that may make one more suitable for your specific use case.