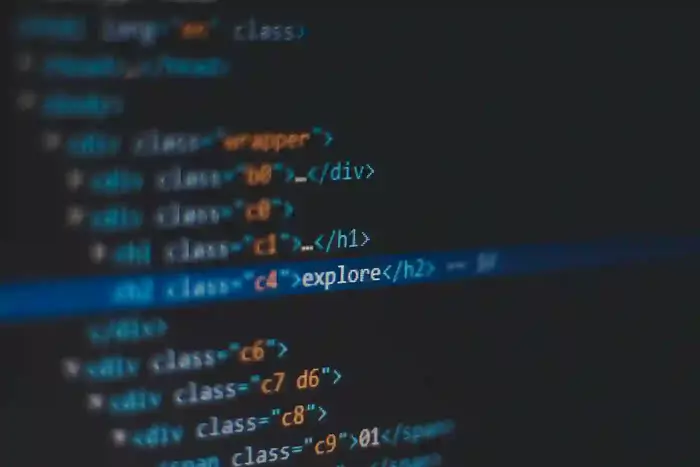
In the world of blockchain technology, Solana is one of the fast-growing ecosystems. It's a high-performance blockchain that can handle thousands of transactions per second with low transaction fees. With the increasing adoption of Solana, developers are building decentralized applications and tools on top of it. One of the essential features of these tools is the ability to fetch transaction data for a given Solana address. In this article, we'll explain how the FlipsideCrypto SDK can be used to fetch transaction data for a Solana address in a NodeJs application.
After creating your project install sdk to your project:
yarn add @flipsidecrypto/sdk
Or if you are using npm
npm install @flipsidecrypto/sdk
Let’s get started:
Import the required dependencies:
import { Flipside, Query, QueryResultSet } from "@flipsidecrypto/sdk"
Note: you may need to change path from
"@flipsidecrypto/sdk"
to
"@flipsidecrypto/sdk/dist/src"
as there is some issue with imports in version 1.1.1.
Initialize `Flipside` with your API key
const flipside = new Flipside(
"<YOUR_API_KEY>",
"https://node-api.flipsidecrypto.com"
);
Getting API key:
To get the api key go to https://sdk.flipsidecrypto.xyz/shroomdk then sign up or login to your account then you can connect your wallet to get the api keys.
Your crypto address on which you want to fetch transaction:
const myAddress = "0x...."
Create a query object for the `query.run` function to execute
const query: Query = {
sql: `SELECT * FROM SOLANA.core.fact_transfers WHERE (tx_from = '${address}' OR tx_to = '${address}')`,
ttlMinutes: 10,
};
Send the `Query` to Flipside's query engine and await the results
const result: QueryResultSet = await flipside.query.run(query);
The Query object contains both the sql and configuration you can send to the query engine for execution. You can find other parameters which you can modify according to your desired output.
type Query = {
// SQL query to execute
sql: string;
// The number of minutes to cache the query results
ttlMinutes?: number;
// An override on the query result cache.
// A value of false will re-execute the query.
cached?: boolean;
// The number of minutes until your query run times out
timeoutMinutes?: number;
// The number of rows to return from the query result set.
// If not specified this defaults to 100k. A max of 1m rows/records
// will be cached and can be paged thru using the pageNumber parameter.
pageSize?: number;
// The page to retrieve cached query results from.
// The default (if not specified) is 1.
pageNumber?: number;
};
In the end this is the hello.ts file that worked for me:
import express, { Request, Response } from "express";
import Cors from "cors";
import { Flipside, Query, QueryResultSet } from "@flipsidecrypto/sdk/dist/src";
const app = express();
const port = 3009;
app.use(
Cors({
origin: "*",
methods: "GET,HEAD,PUT,PATCH,POST,DELETE",
})
);
// add json middleware
app.use(express.json());
app.get("/test", async (req: Request, res: Response) => {
const flipside = new Flipside(
"",
"https://node-api.flipsidecrypto.com"
);
const address =
//blockchain address : you can use one from https://solscan.io/txs
let queryStr = `SELECT * FROM SOLANA.core.fact_transfers WHERE (tx_from = '${address}' OR tx_to = '${address}')`;
const query: Query = {
sql: queryStr,
ttlMinutes: 10,
pageSize: 10,
pageNumber: 1,
};
const result: QueryResultSet = await flipside.query.run(query);
res.status(200).send({
data: result,
});
});
app.listen(port);