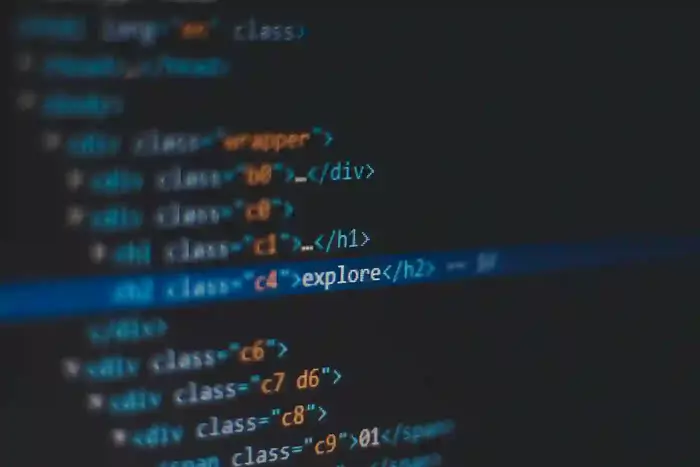
React components can be styled using tagged template literals with the help of the well-known package styled-components. It enables you to write CSS in JavaScript, which can help your code be easier to maintain and more maintainable on certain components. A simple tutorial on creating and utilizing styled components in your React application is provided here:
1.Installation:
If you have not previously, install `styled-components` with React first:
npm install styled-components
2. Import:
Import `styled` from the `styled-components` package at the top of your React
3. Create Styled Components:
Calling the styled function with a tagged template literal as a parameter will produce styled components. You can directly write CSS in your JavaScript code by using the tagged template literal For example, Let's design an easy-to-use button component:
const StyledButton = styled.button`
background-color: #3498db;
color: #fff;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
&:hover {
background-color: #2980b9;
}
`;
4. Use Styled Components:
You can now use your StyledButton component in your JSX just like any other React component:
function MyComponent() {
return (
<div>
<h1>Welcome to my app</h1>
<StyledButton>Click me</StyledButton>
</div>
);
}
5. Global Styles:
You can also use createGlobalStyle from styled-components to apply global styles to your application.
import { createGlobalStyle } from 'styled-components';
const GlobalStyle = createGlobalStyle`
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
}
`;
function App() {
return (
<div>
<GlobalStyle />
{/* The rest of your app */}
</div>
);
}
Reusable, scoped, and responsive styles for your React components are simple to develop with the help of styled-components, which can enhance the readability and maintainability of your code.