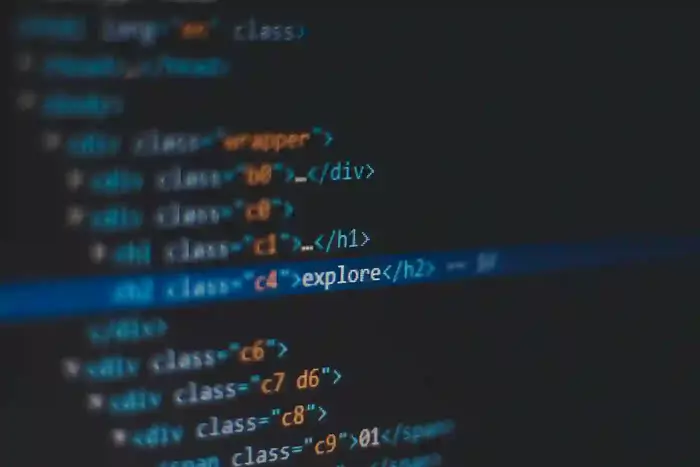
React hooks may be excellent tools for encapsulating and reusing code in your components. The methods below explain how to create your own react hook:
- 1. Your Hook's Name: Pick a memorable name for your unique hook. To identify it as a hook, it should begin with "use" (following the React norm).
- 2. Create a Function: Write the logic of your custom hook in a JavaScript function. If required, this method can utilize other custom hooks in addition to built-in ones like useState and useEffect.
- 3. Define Parameters: If your hook requires any parameters, add them as arguments to your function.
- 4. Return Values: Your components should be able to use the values that the custom hook returns. Anything, including state variables, functions, or both, can be used as these values.
- 5. Example: To handle a counter, a custom hook named useCounter is used in the following straightforward example:
import { useState } from 'react';
function useCounter(initialValue, step) {
const [count, setCount] = useState(initialValue);
const increment = () => {
setCount(count + step);
};
const decrement = () => {
setCount(count - step);
};
return { count, increment, decrement };
}
Now, let's use this custom hook in a component:
import React from 'react';
import useCounter from './useCounter';
function Counter() {
const { count, increment, decrement } = useCounter(0, 1);
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
export default Counter;
In this example:
UseCounter is a custom hook that contains the counter logic. To control the count state and its related functions, Counter is a component that makes use of the useCounter hook. Your component logic can be kept organized and reused across your application by building and utilizing custom hooks.