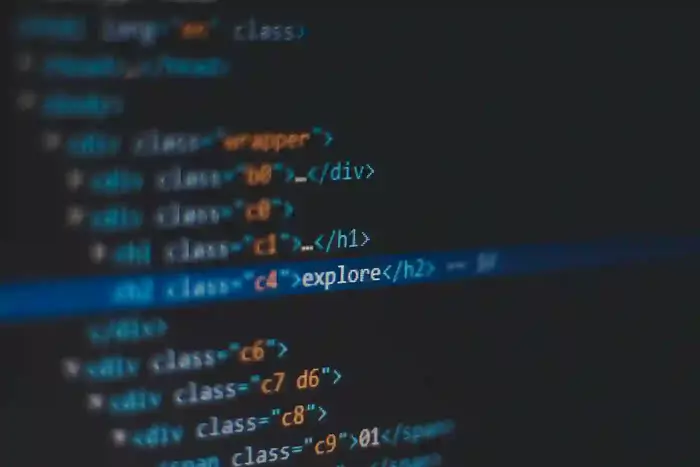
In this article, we are going to explore how we can create a simple TCP socket server and client. For example we will create a simple calculator, where client will ask the server to do the calculation, and server will send the result back to the client.
Setting up project
Create a project folder at your desired location and run the following command in that folder:
npm init -y
Creating a TCP socket server
Create a new file named tcp-socket-server.js:
const net = require("net");
const port = 5000;
const server = net.createServer((socket) => {
console.log("Client connected");
socket.on("data", (data) => {
const strData = data.toString();
console.log(`Received: ${strData}`);
const command = strData.split(",");
const operator = command[0];
const operand1 = parseFloat(command[1]);
const operand2 = parseFloat(command[2]);
let result;
switch (operator) {
case "add":
result = operand1 + operand2;
break;
case "sub":
result = operand1 - operand2;
break;
}
socket.write(result.toString());
});
socket.on("end", () => {
console.log("Client disconnected");
});
socket.on("error", (error) => {
console.log(`Socket Error: ${error.message}`);
});
});
server.on("error", (error) => {
console.log(`Server Error: ${error.message}`);
});
server.listen(port, () => {
console.log(`TCP socket server is running on port: ${port}`);
});
In this code we first imported the net module which is required to create a TCP socket server:
const net = require("net");
Then we created the TCP socket server by calling net.createServer method:
const server = net.createServer((socket) => {
console.log("Client connected");
// event listeners...
});
After that we registered 3 event listeners inside the net.createServer callback function:
- data: Emitted when data is received.
- end: Emitted when the other end of the socket signals the end of transmission.
- error: Emitted when an error occurs.
socket.on("data", (data) => {
const strData = data.toString();
console.log(`Received: ${strData}`);
});
socket.on("end", () => {
console.log("Client disconnected");
});
socket.on("error", (error) => {
console.log(`Socket Error: ${error.message}`);
});
Inside the data event listener we parsed the command that we received from the client and performed the calculation accordingly, then we write the result back to the client by calling socket.write method:
const strData = data.toString();
console.log(`Received: ${strData}`);
const command = strData.split(",");
const operator = command[0];
const operand1 = parseFloat(command[1]);
const operand2 = parseFloat(command[2]);
let result;
switch (operator) {
case "add":
result = operand1 + operand2;
break;
case "sub":
result = operand1 - operand2;
break;
}
socket.write(result.toString());
After that we registered the error event listener on the server object which will be emitted when an error occurs on the server object
server.on("error", (error) => {
console.log(`Server Error: ${error.message}`);
});
At the end we start accepting connections on the specified port by calling the server.listen method:
server.listen(port, () => {
console.log(`TCP socket server is running on port: ${port}`);
});
Now we can start the TCP socket server by running the following command:
node tcp-socket-server.js
Creating a TCP socket client
Create a new file named tcp-socket-client.js:
const net = require("net");
const host = "127.0.0.1";
const port = 5000;
const client = net.createConnection(port, host, () => {
console.log("Connected");
client.write(`sub,95,42.4`);
});
client.on("data", (data) => {
console.log(`Received: ${data}`);
});
client.on("error", (error) => {
console.log(`Error: ${error.message}`);
});
client.on("close", () => {
console.log("Connection closed");
});
In this code we first imported the net module which is required to create a TCP socket connection to the server:
const net = require("net");
Then we created the TCP socket client by calling net.createConnection method and in the callback function we sent the sub command to the server:
const client = net.createConnection(port, host, () => {
console.log("Connected");
client.write(`sub,95,42.4`);
});
After that we registered the data event listener on the client object which will be emitted whenever a client receives data from the server:
client.on("data", (data) => {
console.log(`Received: ${data}`);
});
And after that we registered the error event listener on the client object which will be emitted whenever an error occurs:
client.on("error", (error) => {
console.log(`Error: ${error.message}`);
});
At the end we registered the close event listener on the client object which will be emitted when the socket is fully closed:
client.on("close", () => {
console.log("Connection closed");
});
Now we can start the TCP socket client by running the following command:
node tcp-socket-client.js
After running the TCP socket client, you will see the following output in the client console:
Connected
Received: 52.6
And following output in the server console:
Client connected
Received: sub,95,42.4
I hope this article gives you the basic understanding of how a TCP socket server and client can communicate with each other.
Thanks for reading!