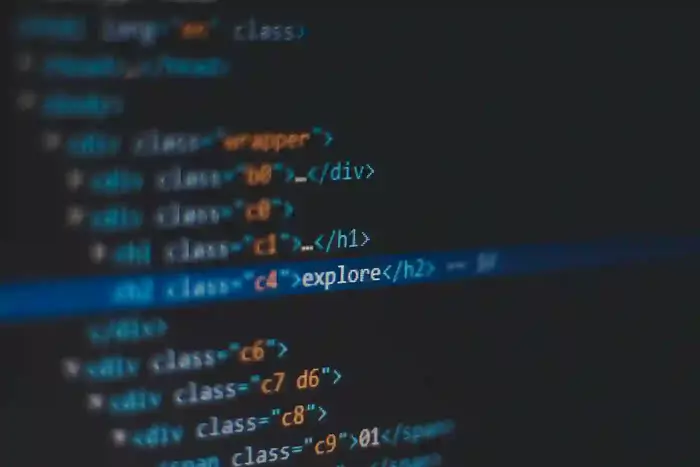
In this article, we will discover how to use Node.js modules in a web browser. We will cover both ways of converting CommonJS and ES6 modules to vanilla javascript.
Setting up project
- Create a project folder at your desired location
- Within the project folder, run the following command
npm init -y
- Install Browserify
npm i -g browserify
- Install md5 package for demo purpose
npm i md5
Converting CommonJS modules to vanilla javascript
- Create a new file named main.js
const md5 = require('md5'); alert(`MD5 generated from index.js: ${md5('1234')}`); global.window.md5 = md5;
- Create one more file named index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Browserify Demo</title> </head> <body> <script src="bundle.js"></script> <script> alert(`MD5 generated from index.html: ${md5('123456')}`); </script> </body> </html>
- Run following command to convert main.js (CommonJS module) to bundle.js (vanilla javascript)
browserify main.js -o bundle.js
Converting ES6 modules to vanilla javascript
- Instead of using require() in main.js we will use import syntax
// main.js import md5 from 'md5'; alert(`MD5 generated from index.js: ${md5('1234')}`); global.window.md5 = md5;
- index.html will remain the same
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Browserify Demo</title> </head> <body> <script src="bundle.js"></script> <script> alert(`MD5 generated from index.html: ${md5('123456')}`); </script> </body> </html>
- Now if we try to run the same command as we did for CommonJS modules we will get the following error:
browserify main.js -o bundle.js
Thats because Browserify does not support import syntax. So we need to convert our ES6 modules to CommonJS modules in order for Browserify to be able to bundle it.
- We need to install few packages to make Browserify understand the ES6 syntax. Run the following command to install required packages
npm i --save-dev @babel/core @babel/preset-env babelify
- Create a new file named babel.config.json and paste following content
{ "presets": ["@babel/preset-env"] }
- Run following command to convert main.js (ES6 module) to bundle.js (vanilla javascript)
browserify main.js -o bundle.js -t babelify
I hope this article solves the mystery of converting CommonJS and ES6 modules to vanilla javascript in order to use it in a web browser.
Thanks for reading!