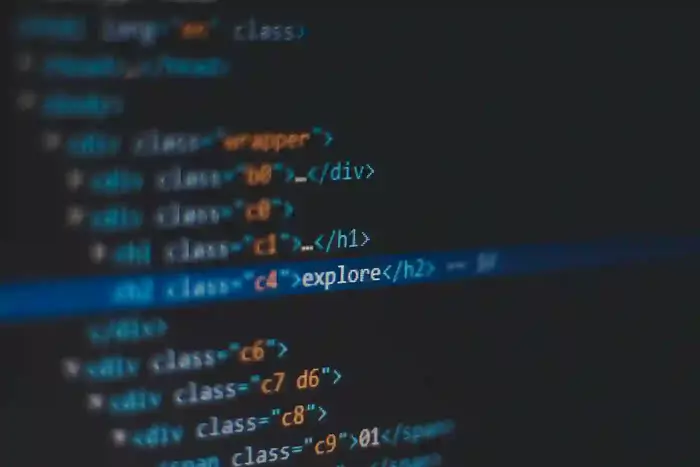
In order to quickly style your HTML or React components without creating unique CSS, Tailwind CSS is a utility-first CSS framework that offers a number of pre-defined utility classes.
There are no additional libraries required to use Tailwind CSS with React components. Take these actions:
Using Create React App or your preferred configuration, start a new React project.
Install the dependencies for Tailwind CSS. Npm or yarn can be used for this:
Using npm:
npm install tailwindcss
Using yarn:
yarn add tailwindcss
Create a Tailwind CSS configuration file. This can be done by using the following command, which creates a tailwind.config.js file in the root directory of your project:
npx tailwindcss init
Add or change the utility classes you want to utilize in tailwind.config.js as necessary.
module.exports = {
theme: {
screens: {
sm: "480px",
md: "768px",
lg: "976px",
xl: "1440px",
},
colors: {
"blue": "#1fb6ff",
"pink": "#ff49db",
"orange": "#ff7849",
"green": "#13ce66",
"gray-dark": "#273444",
"gray": "#8492a6",
"gray-light": "#d3dce6",
},
fontFamily: {
sans: ["Graphik", "sans-serif"],
serif: ["Merriweather", "serif"],
},
extend: {
spacing: {
"128": "32rem",
"144": "36rem",
},
borderRadius: {
"4xl": "2rem",
}
}
}
}
You may import Tailwind CSS right into your React components or your main CSS file:
@import "tailwindcss/base";
@import "tailwindcss/components";
@import "tailwindcss/utilities";
use Tailwind CSS utility and create stunning components
Now Create a Button component
Create a new file called `Button.js` in the `src/components` directory and define your own custom button component:
import React from "react";
const Button = ({ children, onClick, className }) => {
return (
<button
onClick={onClick}
className={`bg-blue-700 hover:bg-blue-500 text-white font-bold py-5 px-5 rounded ${className}`}
>
{children}
</button>
);
};
export default Button;
The text-white, font-bold, py-5, px-5, rounded, hover:bg-blue-500, bg-blue-700 and rounded Tailwind CSS classes are used to decorate the button in this component. In order to enable the insertion of additional custom classes as needed, we additionally use the className prop.
In your App utilize the button component.
import React from "react";
import Button from "./components/Button";
function App() {
return (
<div className="container mx-auto pt-8 text-center">
<h1 className=”font-bold”> Welcome to My First Talwind Integrated App</h1>
<Button onClick={() => alert("Button clicked!")}>Click Me</Button>
Click Me Too
</Button>
</div>
);
}
export default App;
Adding or changing the Tailwind CSS classes in the Button component will allow you to further alter the button styles. You can alter padding, apply hover effects, add box shadows, modify the colors, and many other things. To meet your design needs, Tailwind CSS offers a large selection of utility classes.