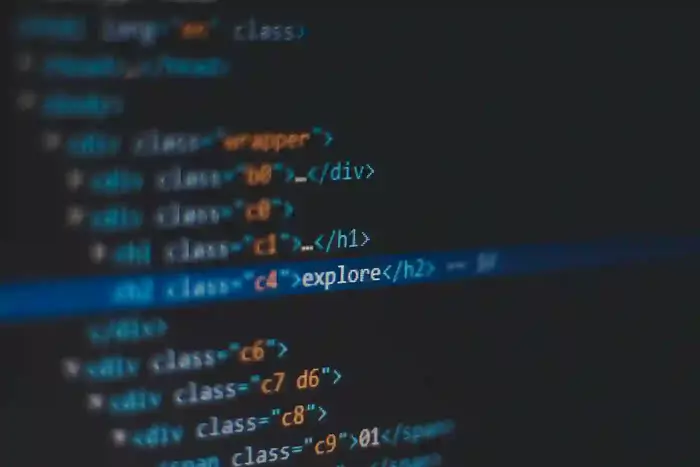
if you're a newbie and want to use React with RTK Query, you're interested in integrate RTK Query into your React application to effectively handle data fetching and state management. A data-fetching and caching package called RTK Query is based on Redux Toolkit.
Here is a step-by-step tutorial for learning React with RTK.
Create a New React Project: Ensure that Node.js and npm (Node Package Manager) are installed on your computer before you begin. Using Create React App:, you may start a new React project.
npx create-react-app my-first-rtk-query-app
cd my-first-rtk-query-app
Install requirements: The next step is to install the RTK Query requirements. Run the following commands inside your project folder in your terminal after opening it:
npm install @reduxjs/toolkit react-redux @reduxjs/toolkit-query react-query
In the src folder, add a new file api.js to the features folder (you can create the folder if it doesn't already exist). Using RTK Query's createApi method, you will specify your API endpoints in this file. For instance:
Create an API Slice:
import { createApi, fetchBaseQuery } from'@reduxjs/toolkit/query/react';
export const api = createApi({
baseQuery: fetchBaseQuery({ baseUrl: '/api' }), // Replace '/api' with your API base URL
endpoints: (builder) => ({
// Define your API endpoints here
getUsers: builder.query({
query: () => 'users', // Replace 'users' with your actual API endpoint
}),
// Add more endpoints as needed
}),
});
Establish the store:
//Import the api object and set the RTK Query middleware parameters in your src/app/store.js file.
import { configureStore } from '@reduxjs/toolkit';
import { api } from '../features/api';
export const store = configureStore({
reducer: {
// Add any other reducers your app may need
[api.reducerPath]: api.reducer,
},
middleware: (getDefaultMiddleware) => getDefaultMiddleware().concat(api.middleware),
});
Use RTK Query Hooks in Components: Now that RTK Query hooks are available, you may use them in your React components to retrieve data from your API. Let's, for instance, retrieve a list of users and display it in a component.
import React from 'react';
import { useGetUsersQuery } from '../features/api';
const UserList = () => {
const { data, error, isLoading } = useGetUsersQuery();
if (isLoading) {
return <div>Loading...</div>; }
if (error) {
return <div>Error: {error.message}</div>;
}
return (
<div>
<h1>User List</h1>
<ul>
{data.map((user) => (
<li key={user.id}>{user.name}</li>
))}
</ul>
</div>
);
};
export default UserList;
The UserList component can be rendered in your main App.js file or any other component.
import React from 'react';
import UserList from './components/UserList';
const App = () => {
return (
<div>
<UserList />
</div>
);
};
export default App;
With this configuration, RTK Query will be used by your React app to manage data fetching and caching, making your data management more effective and simple.